
Methodical 3rd Party Asset Compatibility
Methodical is based on the Microsoft Roslyn, their Open Source Compiler Platform. Roslyn is not compatible with some assets on the Unity Asset Store. The incompatibilities are often easily fixable.
This page is a running list of incompatible assets and fixes. In most cases, getting rid of this error is as easy as patching in exception handling to the asset. If you find an incompatible asset not listed here, please email help@abxygames.com. We’ll promptly help you patch the issue and add the asset to this list.
Other Links
Why does this happen?
Some assets use Reflection to iterate over the types loaded in Unity. This is normally not a problem, but certain DLLs in Roslyn throw an exception when retrieved by Reflection. If an the asset doesn’t catch exceptions properly when iterating through types, you’ll see an error like this:
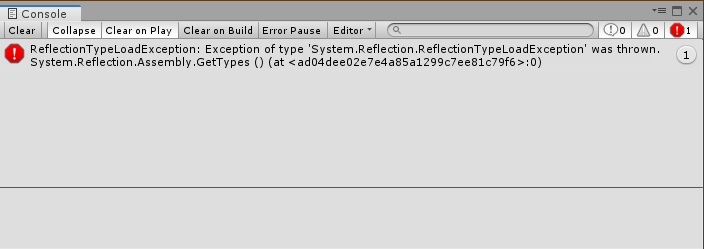
3rd Party Asset List
The following are assets with compatibility issues with Microsoft Roslyn, and their fixes:
Curvy
Location
Assets/Plugins/DevTools/Extensions/Extensions.cs – Line 905
Fix
Change:
public static Type[] GetLoadedTypes()
{
//OPTIM use GetExportedTypes instead of GetTypes?
#if NETFX_CORE
return GetLoadedAssemblies().SelectMany(a => a.DefinedTypes).Select(typeInfo => typeInfo.AsType()).ToArray();
#else
return GetLoadedAssemblies().SelectMany(a => a.GetTypes()).ToArray();
#endif
}
To:
public static Type[] GetLoadedTypes()
{
//OPTIM use GetExportedTypes instead of GetTypes?
#if NETFX_CORE
return GetLoadedAssemblies().SelectMany(a => a.DefinedTypes).Select(typeInfo => typeInfo.AsType()).ToArray();
#else
List<Type> types = new List<Type>();
foreach(Assembly assem in GetLoadedAssemblies())
{
if (assem != null)
{
try
{
Type[] newTypes = assem.GetTypes();
types.AddRange(newTypes);
}
catch (System.Reflection.ReflectionTypeLoadException) { }
}
}
return types.ToArray();
#endif
}
Peek
Peek actually captures exceptions appropriately, but has a debug message that appears when this occurs. Fixing this is as easy as commenting out the message.
Location
Assets\Ludiq\Ludiq.PeekCore\Runtime\Reflection\TypeUtility.cs:887
Fix
Change:
public static IEnumerable<Type> GetTypesSafely(this Assembly assembly)
{
Type[] types;
try
{
types = assembly.GetTypes();
}
catch (Exception ex)
{
Debug.LogWarning($"Failed to load types in assembly '{assembly}'.\n{ex}");
yield break;
}
foreach (var type in types)
{
// Apparently void can be returned somehow:
// http://support.ludiq.io/topics/483
if (type == typeof(void))
{
continue;
}
yield return type;
}
}
To:
public static IEnumerable<Type> GetTypesSafely(this Assembly assembly)
{
Type[] types;
try
{
types = assembly.GetTypes();
}
catch (Exception)
{
//Debug.LogWarning($"Failed to load types in assembly '{assembly}'.\n{ex}");
yield break;
}
foreach (var type in types)
{
// Apparently void can be returned somehow:
// http://support.ludiq.io/topics/483
if (type == typeof(void))
{
continue;
}
yield return type;
}
}